The AI Engineer’s Toolkit: Emerging Tools, Libraries, and Frameworks in 2023-2024
Staying at the cutting edge of AI development requires more than just theoretical knowledge – it demands familiarity with the latest tools reshaping how we build intelligent systems. This article explores 22 emerging technologies that are changing the AI engineering landscape, from computer vision frameworks to agent-based automation systems.
Development Frameworks and Libraries
1. Super Gradients – Production-Ready Computer Vision
What It Is
Super Gradients is a production-ready training library that provides optimized pipelines for computer vision models. Developed by Deci AI, it simplifies the entire workflow from training to deployment with pre-configured recipes for classification, detection, and segmentation tasks.
Key Features
– Production-Ready Implementations: Industry-standard models like YOLO-NAS, YOLO-X, and SegFormer with state-of-the-art performance – Training Efficiency: Built-in distributed training, mixed precision, and hyperparameter optimization – Deployment Pipeline: Streamlined export to ONNX, TensorRT, and other production formats – Pre-trained Models: Extensive collection of weights trained on standard datasets
Code Example
# Training a custom object detection model with Super Gradients
from super_gradients.training import models, Trainer
from super_gradients.training.dataloaders.dataloaders import (
get_classification_dataloaders,
get_detection_dataloaders
)from super_gradients.training.metrics import DetectionMetrics
from super_gradients.training.losses import PPYoloELoss
# Initialize trainer
= Trainer(experiment_name="my_object_detector", ckpt_root_dir="checkpoints/")
trainer
# Create data loaders with proper COCO dataset
= get_detection_dataloaders(
train_loader, val_loader ="coco_detection_yolo_format",
dataset_name="path/to/coco",
data_dir="train2017",
train_dir="val2017",
val_dir="annotations/instances_train2017.json",
train_json_file="annotations/instances_val2017.json",
val_json_file=16,
batch_size=4
num_workers
)
# Initialize model with pre-trained weights
= models.get("yolox_s", pretrained_weights="coco")
model
# Define training parameters
= {
train_params "max_epochs": 10,
"lr_mode": "cosine",
"initial_lr": 0.001,
"warmup_epochs": 1,
"loss": PPYoloELoss(),
"metrics": [DetectionMetrics(post_prediction_callback=None, score_thres=0.1)],
"optimizer": "Adam",
"mixed_precision": True,
}
# Train the model
trainer.train(=model,
model=train_params,
training_params=train_loader,
train_loader=val_loader
valid_loader )
2. GPT Engineer – From Prompt to Functional Application
What It Is
GPT-Engineer is an open-source project that leverages large language models to generate entire codebases from natural language prompts. It bridges the gap between idea and implementation, handling everything from system architecture to API design to user interfaces.

Key Features
– Holistic Code Generation: Creates a complete, structured project rather than just code snippets – Iterative Refinement: Converses with you to understand requirements and make adjustments – Language and Framework Flexibility: Works across multiple programming languages and frameworks – Project Organization: Generates proper file structure, dependencies, and documentation
Workflow
1. Specification: Write natural language requirements in an instructions.md
file 2. Generation: GPT-Engineer generates a complete codebase with proper architecture 3. Refinement: Iteratively provide feedback to improve and extend the implementation 4. Execution: Run the generated code with minimal manual intervention
Use Cases
– Rapid prototyping of web applications and APIs – Creating MVPs for startups and new projects – Learning new frameworks by examining generated implementations – Automating boilerplate code generation
3. Chainlit – The Streamlit for LLM Applications
What It Is
Chainlit is a Python framework for building conversational AI interfaces with the same simplicity that Streamlit brought to data visualization apps. It enables rapid prototyping of language model applications with built-in UI components specifically designed for conversational experiences.
Key Features
– Chat UI Components: Pre-built elements for messages, feedback, file uploads, and more – LangChain Integration: Seamless compatibility with LangChain’s agents, chains, and tools – Message Tracing: Visualizes the execution flow of complex LLM-based systems – Multi-modal Support: Handles text, images, and other data types within the conversation – Cloud Deployment: Easy deployment options to share your applications
Code Example
# Creating a simple conversational app with Chainlit
import chainlit as cl
from langchain.chat_models import ChatOpenAI
from langchain.schema import HumanMessage, SystemMessage
= ChatOpenAI(model="gpt-3.5-turbo", temperature=0.7)
llm
@cl.on_chat_start
async def start():
# Send a welcome message
await cl.Message(
="Welcome to Tech Assistant! Ask me anything about programming."
content
).send()
# Store the system message in user session
set(
cl.user_session."system_message",
="You are a helpful programming assistant.")
SystemMessage(content
)
@cl.on_message
async def main(message: str):
# Get the system message
= cl.user_session.get("system_message")
system_message
# Create a list of messages
= [system_message, HumanMessage(content=message)]
messages
# Call the LLM
= await llm.agenerate([messages])
response = response.generations[0][0].text
response_message
# Send the response
await cl.Message(content=response_message).send()
Getting Started
pip install chainlit langchain openai
chainlit run app.py
AI Agents and Automation
4. App Agent – LLM-Powered Mobile Automation
What It Is
App Agent represents an emerging category of tools that use large language models to control mobile devices through natural language instructions. These systems bridge the gap between human intent and mobile OS APIs, enabling hands-free automation of complex tasks.
Key Capabilities
– Natural Language Control: Convert everyday instructions into precise mobile OS commands – UI Navigation: Intelligently interact with app interfaces without hardcoded element selectors – Cross-App Workflows: Chain actions across multiple applications (e.g., “take a photo and share it via email”) – Adaptive Learning: Improve execution by remembering successful interaction patterns
Potential Applications
– Accessibility: Enable voice-controlled operation for users with limited mobility – Test Automation: Generate and run test scenarios across mobile applications – Personal Productivity: Automate repetitive tasks on smartphones and tablets – Customer Support: Guide users through complex procedures remotely
Implementation Approaches 1. Vision-Language Models: Using screen captures to understand UI state and guide interaction 2. API Integration: Direct access to app functions through predefined interfaces 3. Hybrid Systems: Combining visual understanding with API-based execution for reliability
5. vimGPT – Browser Automation Through Natural Language
What It Is
vimGPT represents a new paradigm in browser automation that combines the efficiency of Vim-like commands with the flexibility of natural language processing. Unlike traditional automation tools that require scripting, vimGPT allows users to control web browsers through concise, intuitive instructions processed by large language models.
Key Features
– Prompt-Driven Automation: Control browser actions through natural language instructions – Context Awareness: Understands the current state of webpages to make intelligent decisions – Pattern Recognition: Identifies UI elements without requiring explicit selectors – Command Chaining: Executes complex sequences of actions from a single instruction
Practical Applications
– Data Collection: Extract information from websites without writing custom scrapers – Form Automation: Fill out complex forms with minimal instruction – Workflow Automation: Automate repetitive web-based tasks in business processes – Testing: Generate and execute test cases for web applications
Current Implementations
While “vimGPT” specifically may be an emerging concept, similar capabilities are being developed in projects like: – BrowserGPT – Selenium + GPT integrations – Playwright with LLM control layers
6. GPT Crawler – Intelligent Web Knowledge Base Builder
What It Is
GPT Crawler is a specialized web scraping framework designed for creating high-quality knowledge bases for AI applications. Unlike traditional scrapers, it uses language models to intelligently navigate websites, extract relevant information, and organize content for retrieval-augmented generation (RAG) systems.
Key Features
– Semantic Navigation: Understands website structure and content relationships – Content Filtering: Distinguishes between valuable information and boilerplate – Metadata Extraction: Captures publication dates, authors, categories, and other context – Knowledge Organization: Structures extracted data into interconnected knowledge graphs – Vector Embedding: Processes text into embedding formats ready for semantic search
Workflow 1. Seed URLs: Provide starting points for crawling 2. Intelligent Traversal: Language model determines which links to follow based on content relevance 3. Content Extraction: Clean, meaningful text is separated from navigation and advertisements 4. Processing Pipeline: Raw content is transformed into structured data, chunked, and embedded 5. Knowledge Base Integration: Results are stored in vector databases for RAG applications
Retrieval and Search Systems
7. RAGraville – Advanced Retrieval for LLM Applications
What It Is
RAGraville represents a suite of cutting-edge techniques for Retrieval-Augmented Generation (RAG) that improve upon basic vector search methods. It integrates ColBERT (Contextualized Late Interaction BERT) and other state-of-the-art retrieval models to enable more precise and nuanced information retrieval for language model applications.

Key Innovations
– Late Interaction Architecture: Unlike single-vector representations, ColBERT preserves token-level information for more precise matching – Multi-Vector Encoding: Represents documents as collections of vectors rather than single points in embedding space – Hybrid Retrieval: Combines sparse (keyword) and dense (semantic) methods for better recall – Re-ranking Pipelines: Two-stage retrieval with broad candidate generation followed by precise ranking
Performance Benefits
– Higher Precision: More accurate document retrieval, especially for complex or ambiguous queries – Better Context Selection: Identifies the most relevant passages within documents – Reduced Hallucination: More reliable factual grounding for language model responses – Support for Longer Contexts: Efficiently handles documents with thousands of tokens
Implementation Stack – Embedding Models: Specialized retrievers like ColBERT, E5, or BGE – Vector Databases: Pinecone, Weaviate, or Qdrant with multi-vector support – Re-ranking Models: Cross-encoders for candidate refinement – Orchestration Layer: Managing the multi-stage retrieval process
8. AI Agent Search / SciPhi Search
What It Is
AI Agent Search represents a paradigm shift from passive search engines to active research assistants. These systems use language models as reasoning agents to decompose complex queries, strategically gather information, synthesize findings, and present coherent answers. SciPhi Search specifically focuses on scientific and philosophical domains where nuanced understanding is essential.
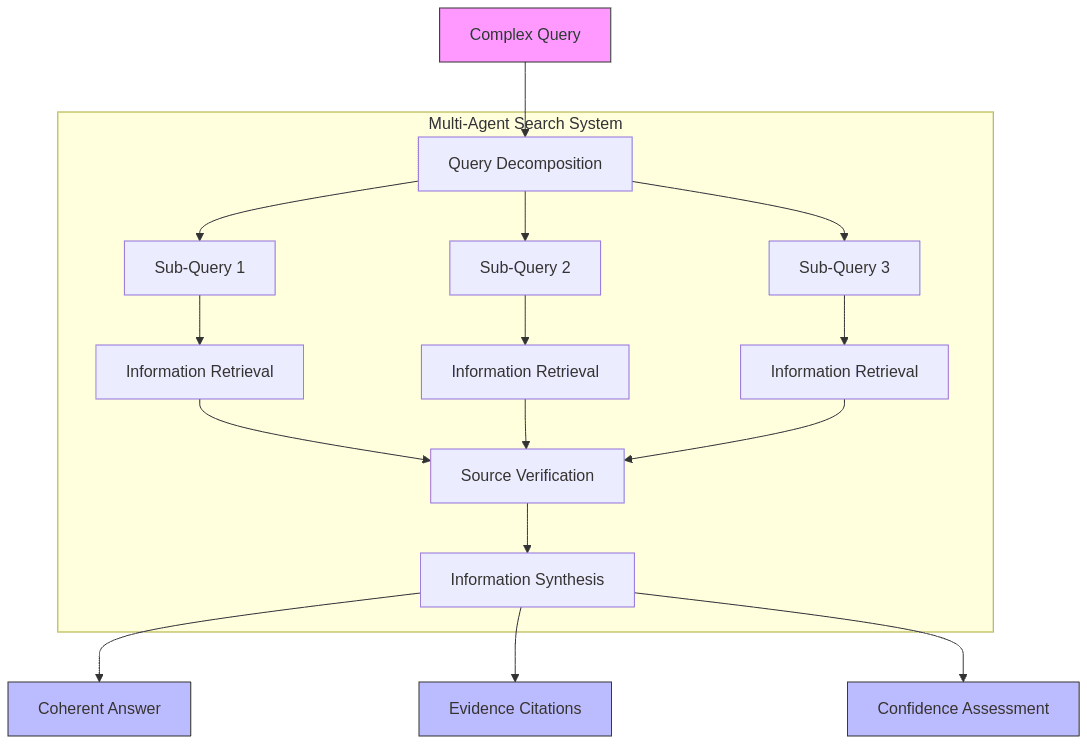
Key Capabilities
– Query Decomposition: Breaking complex questions into manageable sub-queries – Strategic Retrieval: Using different search strategies based on the type of information needed – Source Triangulation: Comparing information across multiple sources to verify accuracy – Knowledge Synthesis: Combining fragments of information into coherent explanations – Reasoning Transparency: Explaining the search and synthesis process
Architecture Components
– Planning Layer: Determines how to approach complex information needs – Retrieval Layer: Executes searches across multiple knowledge sources – Evaluation Layer: Assesses relevance and reliability of retrieved information – Synthesis Layer: Combines information into comprehensive answers – Explanation Layer: Documents the research process and reasoning
Applications
– Academic Research: Literature review and hypothesis exploration – Evidence-Based Decision Making: Gathering and analyzing information for policy or business decisions – Educational Support: Providing comprehensive explanations of complex topics – Fact-Checking: Verifying claims against reliable sources
Audio and Speech Technologies
9. Lyra – Advanced Audio Processing From Google
What It Is
Lyra was initially developed by Google as an ultra-low-bitrate speech codec for bandwidth-constrained environments. While the original Lyra focused on compression, Google has expanded its audio AI portfolio with generative models like MusicLM and AudioLM that can create novel audio content from textual descriptions.
Key Components
– Neural Vocoder: Lyra’s core technology reconstructs speech from minimal data – Audio Generation: Related Google projects synthesize music and environmental sounds – Speech Enhancement: Noise reduction and clarity improvements for communications – Cross-Modal Translation: Converting between text, music notation, and audio
Applications
– Low-Bandwidth Communication: High-quality voice calls over extremely limited connections – Content Creation: Generating custom music and sound effects for media projects – Accessibility: Converting text to natural-sounding speech for screen readers – Audio Restoration: Recovering and enhancing degraded audio recordings
Developer Resources
– Lyra GitHub Repository – AudioLM Research – MusicLM Examples
10. WhisperSpeech – End-to-End Speech Processing
What It Is
WhisperSpeech extends the capabilities of OpenAI’s Whisper speech recognition model to create a comprehensive speech processing pipeline. While Whisper itself focuses on speech-to-text transcription, WhisperSpeech adds text-to-speech capabilities and intermediate processing to enable complete audio workflows.
Key Features
– High-Quality Transcription: State-of-the-art speech recognition across multiple languages – Neural Text-to-Speech: Natural-sounding voice synthesis with prosody control – Voice Cloning: The ability to reproduce distinctive vocal characteristics – Translation Pipeline: End-to-end speech translation between languages – Audio Editing: Text-based modification of speech recordings
Technical Architecture
– Encoder-Decoder Design: Converts between audio and textual representations – Fine-Tuning Options: Adaptation to specific domains or accents – Real-Time Processing: Optimized for low-latency applications – Multimodal Integration: Combines with visual or contextual information
Implementation Example
# Conceptual end-to-end pipeline using Whisper and TTS components
import whisper
import torch
from transformers import SpeechT5Processor, SpeechT5ForTextToSpeech
from datasets import load_dataset
# Initialize models
= whisper.load_model("large")
transcriber = SpeechT5Processor.from_pretrained("microsoft/speecht5_tts")
processor = SpeechT5ForTextToSpeech.from_pretrained("microsoft/speecht5_tts")
tts_model
# Load voice embedding for TTS
= load_dataset("Matthijs/cmu-arctic-xvectors", split="validation")
embeddings_dataset = torch.tensor(embeddings_dataset[7306]["xvector"]).unsqueeze(0)
speaker_embeddings
# Speech-to-text
= "meeting_recording.wav"
audio_path = transcriber.transcribe(audio_path)["text"]
transcript
# Process text (e.g., summarize, translate)
= process_text(transcript) # Placeholder for text processing
summary
# Text-to-speech
= processor(text=summary, return_tensors="pt")
inputs = tts_model.generate_speech(
speech "input_ids"],
inputs[
speaker_embeddings,=None # Will use default vocoder
vocoder
)
# Save the result
import soundfile as sf
"meeting_summary.wav", speech.numpy(), samplerate=16000) sf.write(
Content Generation and Creative Tools
11. Rosebud.ai – AI-Accelerated Game Development
What It Is
Rosebud.ai provides an integrated suite of generative AI tools designed specifically for game development. It enables creators to rapidly generate and iterate on game assets, characters, environments, and narratives without requiring extensive artistic or programming expertise.
Key Capabilities
– Character Generation: Create detailed 3D character models from text descriptions – Environment Design: Generate landscapes, buildings, and interiors based on thematic inputs – Texture Creation: Produce high-quality textures and materials for 3D assets – Narrative Development: Generate storylines, dialogue, and quest structures – Animation Assistance: Help with character animations and movements
Game Development Workflow Integration
– Unity & Unreal Engine Plugins: Direct integration with popular game engines – Asset Pipeline Compatibility: Outputs compatible with standard game development tools – Version Control: Tracking iterations of AI-generated content – Collaborative Features: Shared workspaces for development teams
Impact on Development Process
– Rapid Prototyping: Test gameplay concepts with placeholder assets in hours instead of days – Indie Empowerment: Enables small teams to create content at scale – Iteration Speed: Quick generation of alternatives for creative decision-making – Focus Shift: Developers can concentrate on gameplay mechanics while AI handles asset creation
12. Sora – Advanced Multilingual OCR System
What It Is
Sora, in this context, refers to a sophisticated optical character recognition (OCR) toolkit specialized in extracting text from images and documents across multiple languages and scripts. (Note: This is distinct from OpenAI’s Sora video generation model released in 2024). This OCR system combines computer vision with language understanding to handle complex layouts, mixed languages, and imperfect source materials.
Key Features
– Script Versatility: Recognizes Latin, Cyrillic, Arabic, CJK (Chinese, Japanese, Korean), and other writing systems – Layout Analysis: Understands document structure including columns, tables, and text flow – Context-Aware Recognition: Uses surrounding content to improve accuracy – Handwriting Support: Processes both printed and handwritten text – Post-Processing Intelligence: Corrects recognition errors using language models
Technical Foundation
– Vision Transformers: For understanding document structure and visual context – Language-Specific Models: Specialized for particular writing systems – Layout-Aware Design: Preserves document structure in extracted text – Integration Capabilities: APIs for incorporation into document processing pipelines
Application Areas
– Document Digitization: Converting paper archives to searchable digital formats – Global Compliance: Processing international regulatory documents – Research Support: Extracting text from multilingual academic materials – Historical Preservation: Digitizing manuscripts and historical texts
Developer Tools and Utilities
13. Guidance – Structured Programming for Language Models
What It Is
Guidance is a programming paradigm developed by Microsoft that brings software engineering principles to prompt engineering. It provides a structured way to control language model generation through templates with explicit control flow, constraints, and validation.
Key Features
– Template System: Define generation patterns with explicit placeholders – Control Flow: Include conditionals, loops, and recursion in LLM execution – Constraints: Apply regex patterns and validation rules to outputs – Composability: Build complex prompts from reusable components – Interactive Generation: Stream results with the ability to guide generation in progress
Code Example
import guidance
# Define a model to use
= guidance.llms.OpenAI("gpt-3.5-turbo")
model
# Create a structured extraction template
= guidance("""
expert_analysis {{#system~}}
You are a cybersecurity expert analyzing potential vulnerabilities.
{{~/system}}
{{#user~}}
Please analyze this code snippet for security issues:
```python
{{code}}
{{~/user}}
{{#assistant~}} ## Security Analysis
{{#each gen “issues” num_items=3 temperature=0.7}} ### Issue {{@index + 1}}: {{gen “title” temperature=0.2 max_tokens=10}} Severity: {{select “severity” options=[“Low”, “Medium”, “High”, “Critical”]}} Description: {{gen “description” temperature=0.5 max_tokens=100}} Recommendation: {{gen “recommendation” temperature=0.5 max_tokens=100}}
{{/each}} {{~/assistant}} “““)
Run the template with input
result = expert_analysis( code=““” def authenticate(username, password): if username == “admin” and password == “password123”: return True return False ““” )
print(result) “`
Benefits Over Traditional Prompting
– Predictability: More consistent outputs through structured generation – Transparency: Clear visualization of the prompt structure and generation process – Efficiency: Reduced token usage through targeted generation – Maintainability: Easier to debug and update complex prompting logic
14. vrm.asmirnov.xyz – VRAM Usage Estimator
What It Is
This tool provides accurate estimates of GPU VRAM requirements for AI model training and inference. It helps developers and researchers determine whether their hardware can handle specific model configurations before committing resources to deployment or training.
Key Features
– Model Architecture Analysis: Calculates memory needs based on model architecture – Training Configuration: Accounts for batch size, precision format, and optimizer state – Inference Profiling: Estimates deployment requirements including throughput considerations – Hardware Compatibility: Matches requirements to specific GPU models and configurations
Calculation Factors
– Model Parameters: Base memory for weights and biases – Activation Maps: Memory for intermediate outputs during forward pass – Gradients: Memory for backpropagation – Optimizer States: Additional memory for optimizer variables (Adam, AdamW, etc.) – Batch Size Impact: Linear scaling with input batch dimensions – Precision Format: FP32 vs. FP16 vs. INT8 quantization differences
Practical Applications
– Hardware Planning: Determining GPU requirements for new projects – Cost Optimization: Avoiding overprovisioning of cloud GPU resources – Configuration Tuning: Finding optimal batch sizes and precision formats – Model Architecture Decisions: Evaluating memory impacts of design choices
15. Microsoft Sophia – Enterprise AI Assistant
What It Is
Microsoft Sophia represents an evolution of business-focused AI assistants, building on the foundation of Microsoft Copilot but with enhanced capabilities for enterprise workflows. It integrates deeply with the Microsoft 365 ecosystem to provide contextual assistance across business applications.
Key Capabilities
– Document Intelligence: Analyzes and summarizes business documents with domain awareness – Meeting Assistant: Participates in and summarizes meetings with action item extraction – Data Analysis: Generates insights from business data in Excel and Power BI – Process Automation: Creates and maintains workflows across Microsoft applications – Knowledge Management: Organizes and retrieves information from corporate knowledge bases
Integration Points
– Microsoft 365: Word, Excel, PowerPoint, Outlook, Teams – Dynamics 365: CRM and ERP systems – Power Platform: Power Automate, Power Apps, Power BI – Azure Services: Cloud computing and storage infrastructure
Enterprise-Grade Features
– Security Compliance: Respects organizational data boundaries and access controls – Audit Tracking: Records AI assistant actions for compliance purposes – Domain Adaptation: Customizable to specific industry terminology and workflows – Private Deployment: Options for data sovereignty and privacy requirements
Building Blocks and Infrastructure
16. With Martian – Intelligent LLM Routing Platform
What It Is
“With Martian” describes an intelligent routing layer for large language model applications. It directs incoming requests to the most appropriate LLM based on task requirements, cost considerations, and performance characteristics, enabling multi-model strategies without complex integration work.

Key Features
– Cost Optimization: Routes simple queries to affordable models, complex ones to advanced models – Specialized Routing: Directs domain-specific questions to models with relevant expertise – Performance Monitoring: Tracks response quality and latency across different providers – Fallback Mechanisms: Handles API failures by redirecting to alternative models – Request Transformation: Optimizes prompts for each target model’s specific capabilities
17. Party Rock – AWS Low-Code LLM Application Builder
What It Is
Party Rock is Amazon Web Services’ platform for rapidly creating and deploying LLM-powered applications without extensive coding. It provides a visual interface and pre-built templates for common AI use cases, allowing developers to quickly prototype and deploy generative AI solutions on AWS infrastructure.
Key Features
– Visual Builder: Drag-and-drop interface for application design – Pre-built Templates: Starting points for common use cases like chatbots and content generators – AWS Integration: Native connections to Amazon Bedrock, Lambda, API Gateway, and other services – Customization Options: Extension points for developers to add custom logic – One-Click Deployment: Streamlined process for moving from prototype to production
Supported Use Cases
– Conversational Interfaces: Chatbots and virtual assistants – Content Generation: Text, image, and multimedia creation tools – Data Analysis: Insights extraction from structured and unstructured data – Process Automation: Workflow automation with natural language triggers
Deployment Model – Serverless Architecture: Scales automatically with usage – AWS Security Model: Inherits AWS IAM permissions and security controls – Monitoring Integration: Connected to CloudWatch for performance tracking – Cost Management: Pay-per-use model with usage controls
18. phidata – AI Application Development Framework
What It Is
phidata is an opinionated framework for building production-ready AI applications with a focus on data processing pipelines, workflow orchestration, and model deployment. It streamlines the transition from experimental notebooks to robust, scalable applications.
Key Features
– Workflow Orchestration: Define and run complex AI pipelines with dependencies – Data Processing: Standardized interfaces for ETL operations and feature engineering – Model Integration: Seamless connection to training and inference workflows – Deployment Templates: Production-ready configurations for various environments – Monitoring Hooks: Built-in observability for pipeline performance
Component Architecture
– Workflows: Define processing steps and their relationships – Resources: Declare infrastructure and dependencies – Executors: Run code in various environments (local, container, serverless) – Storage: Manage data and artifacts across the pipeline – ML Operations: Train, evaluate, and deploy models
Development Experience
– Python-First: Native Python API without complex configuration formats – Local Development: Test full pipelines on local machines before deployment – Reproducibility: Deterministic execution with version control – Incremental Adoption: Can be integrated into existing projects gradually
Experimental and Research Tools
19. Websight – Visual to HTML Conversion
What It Is
Websight is a Hugging Face-hosted tool that transforms screenshots or design mockups into functional HTML and CSS code. It leverages computer vision and generative models to analyze visual layouts and reproduce them as web code, bridging the gap between design and implementation.
Key Capabilities
– Layout Recognition: Identifies structural elements like headers, navigation, content areas – Component Detection: Recognizes common UI components (buttons, forms, cards) – Style Extraction: Analyzes colors, typography, and spacing – Responsive Consideration: Generates code with mobile adaptability in mind – Framework Options: Can target plain HTML/CSS or popular frameworks like Bootstrap or Tailwind
Workflow Integration
– Design Handoff: Accelerates the transition from design tools to development – Prototyping: Quickly turns concept sketches into interactive prototypes – Legacy Conversion: Transforms screenshots of existing sites for modernization – Accessibility Enhancement: Adds proper semantic markup during conversion
Accuracy Considerations
– Simple Layouts: High fidelity for standard patterns and components – Complex Interactions: May require manual refinement for advanced behaviors – Custom Elements: Novel UI components might need adjustment – Responsive Design: Generated code provides a starting point for responsiveness
20. Haven.run – Experimental AI Environment Platform
What It Is
Haven.run appears to be an experimental platform for AI experiment tracking and environment management. While potentially not actively maintained (“seems old”), it represents an important category of tools focused on reproducibility and collaboration in AI research.
Potential Features
– Experiment Tracking: Recording hyperparameters, metrics, and results – Environment Isolation: Containerized execution environments for reproducibility – Artifact Management: Version control for datasets and model weights – Visualization Tools: Interactive dashboards for experiment results – Collaboration Features: Sharing experiments and results with team members
Modern Alternatives
For production use, consider more actively maintained alternatives: – Weights & Biases – MLflow – DVC – Neptune.ai
21. Robust AI – Secure and Trustworthy AI Systems
What It Is
Robust AI encompasses a collection of techniques and practices for building AI systems that are secure, reliable, and resistant to attacks or manipulation. This field focuses on addressing vulnerabilities unique to AI systems, from data poisoning to adversarial examples to prompt injection.
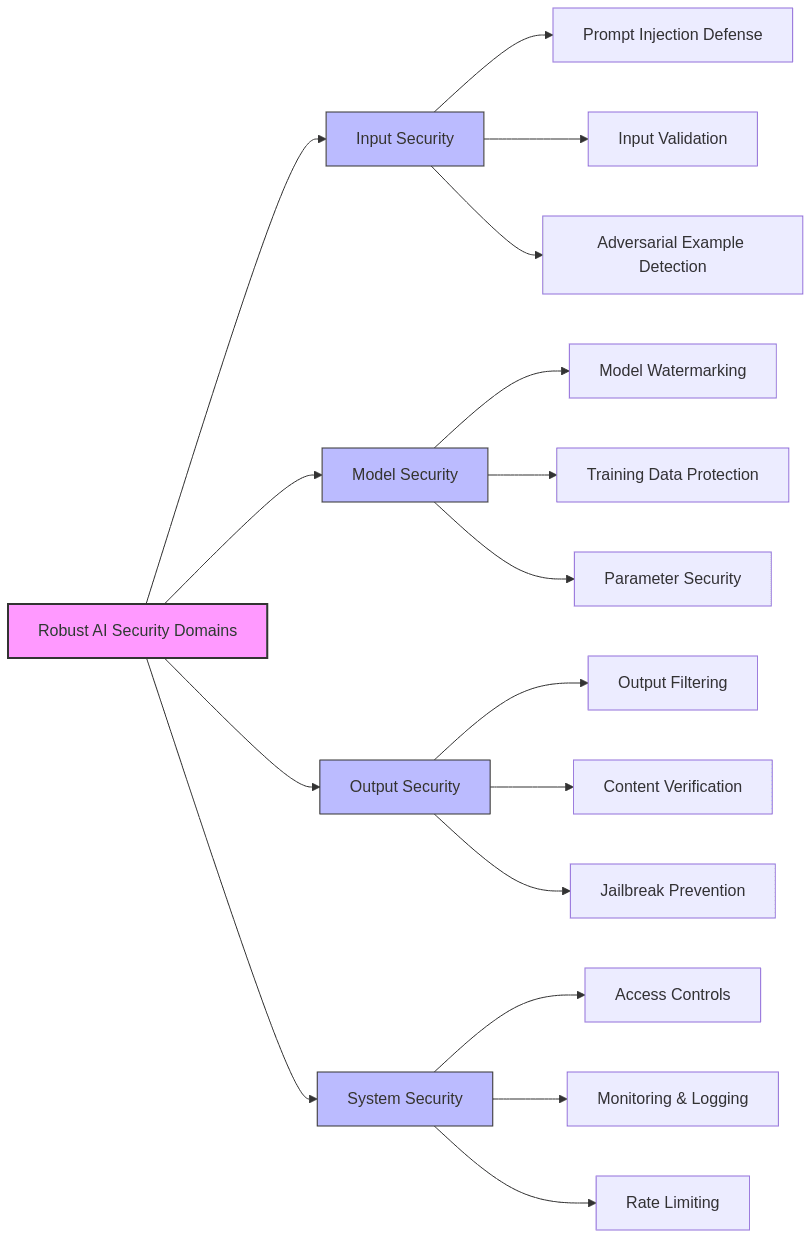
Key Security Domains
– Adversarial Robustness: Defending against inputs designed to fool models – Prompt Security: Preventing injection attacks and prompt leakage – Data Poisoning Defense: Detecting and mitigating training data manipulation – Privacy Preservation: Protecting sensitive information in training data and outputs – Explainability: Making model decisions interpretable for security auditing
Implementation Approaches
– Adversarial Training: Exposing models to attack examples during training – Input Validation: Sanitizing and validating inputs before processing – Output Filtering: Scanning generated content for security issues – Monitoring Systems: Detecting unusual patterns in model behavior – Formal Verification: Mathematical guarantees of model properties
Industry Applications
– Finance: Secure AI for fraud detection and risk assessment – Healthcare: Protecting patient data in medical AI applications – Critical Infrastructure: Reliable AI for power grids, transportation systems – Content Moderation: Manipulation-resistant content filtering
22. typeset.po – Specialized Document Processing
What It Is
“typeset.po” likely refers to a specialized tool combining document typesetting (similar to LaTeX) with internationalization capabilities (the .po file extension is commonly used for translation files). This tool would enable multilingual document preparation with consistent formatting across languages.
Potential Features
– Multilingual Typesetting: Handles right-to-left scripts, CJK characters, and other language-specific requirements – Translation Management: Integrates with localization workflows – Template System: Maintains consistent document structure across translations – Publishing Pipeline: Generates PDFs, web content, and other formats – Collaborative Editing: Supports multiple contributors working on different language versions
Possible Use Cases
– Technical Documentation: Maintaining product documentation in multiple languages – Academic Publishing: Preparing multilingual research papers – Legal Documents: Creating contracts and agreements for international use – Educational Materials: Developing learning resources for global audiences
Conclusion: The Evolving AI Engineering Landscape
The tools and frameworks highlighted in this article represent the cutting edge of AI engineering in 2023-2024. They demonstrate several key trends shaping the field:
-
Abstraction and Accessibility: Frameworks like Super Gradients, GPT Engineer, and Chainlit are making advanced AI capabilities accessible to developers without requiring deep expertise in the underlying models.
-
Agent-Based Architectures: Tools like App Agent, vimGPT, and AI Agent Search point to a future where AI systems act as autonomous agents rather than passive tools, understanding context and taking initiative.
-
Enhanced Retrieval Systems: Advanced RAG implementations like RAGraville are moving beyond simple vector search to provide more accurate and nuanced information retrieval for knowledge-intensive applications.
-
Multimodal Integration: Solutions spanning text, audio, vision, and interaction (WhisperSpeech, Websight, Rosebud.ai) demonstrate the industry’s move toward seamless integration across modalities.
-
Production Focus: Many of these tools prioritize the transition from experimental AI to production systems, addressing deployment, security, and scalability challenges.
As an AI engineer or researcher, staying familiar with this evolving toolkit is essential. The pace of innovation means that new capabilities emerge weekly, often transforming what’s possible in AI application development. By monitoring these emerging tools and participating in their open-source communities, you can ensure your projects leverage the best available technologies while contributing to the field’s advancement.
Which of these technologies are you most excited to explore? Share your experiences and questions in the comments below.